Struggling to verify the input field functioning with Selenium? Don’t panic! Selenium comes with dedicated strategies that help you input data into various fields to verify its proper functioning. It means that even if you are using advanced technologies like the Selenium ChromeDriver to implement automated cross-browser testing, you can ensure that all your input fields are working properly.
Do not know how? We’re here for you! Our article will help you understand everything that you need to know about typing into input fields with Selenium. We’ll go through the syntax, real-world scenarios, best practices, and some of the common challenges that we can face in this process. While discussing the challenges, we’ll also list out the common solutions for them.
What Are Input Fields
Input fields, which are commonly defined with the tags <input>, <textarea>, and <contenteditable> tags in HTML, are designed to capture various user inputs for performing tasks and showing different elements of the application. These fields can store some of the most common information, like text, password, e-mail, and search results.
To help you understand further, if you want to store a username as an input field on your application, you will have to use the code:
<input type=”text” id=”username” name=”username”>
Now, while you are implementing automation testing with Selenium, you will have access to various methods and features that can interact with these elements using automation test scripts. During the interaction process, these scripts will mimic various user behaviors to understand how these fields function when they are exposed to various real-world parameters.
Selenium’s Core Methods for Typing
Let us now divert our attention to some of the core features that are natively available with Selenium. These methods will help you type into your input fields while executing automation testing:
sendKeys()
The sendKeys() method is one of the most commonly used methods that you can use to perform typing. With this method, you can simulate various forms of keystrokes in multiple input fields. For example, if you are asking the users to provide input in the following format:
WebElement inputField = driver.findElement(By.id(“username”));
inputField.sendKeys(“testuser”);
You have to create a Python-based test script like the one given below to use this method for typing within the field:
input_field = driver.find_element(By.ID, “username”)
input_field.send_keys(“testuser”)
clear()
If you are typing into certain input fields that have prefilled data, there is a high possibility of data duplication. How do you deal with such a scenario? It’s very simple! You have to use the clear() method before sending any new input within the field. How do you implement that? The following sample code will help you understand the implementation of this method:
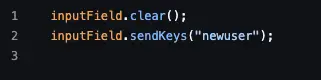
Locating Input Fields
Although we have covered the various native input fields that will be available to you while using Selenium, one of the common questions that might arise in your mind is how we can even test the input fields without even locating them first. To help you in this segment, let us divert our attention towards the popular locator strategies available with Selenium:
- The simplest way is to locate these elements on your web application by using their ID. This is a very common way of locating strategy because, in most cases, every element has a unique ID, so there is no possibility of data clash.
- You can also locate an element by using its name. However, while using this attribute, you need to be very cautious, as in modern applications, various elements can share the same name or at least a part of it. A great practice, in this regard, would be to ask your developers to name the elements depending on the purpose they serve.
- You can also locate an element by referring to its class name. It is a very common practice, as class names tend to be unique depending on the section of the application.
- Selenium also allows you to implement advanced locator strategies like CSS selectors and XPath for element location. The most important benefit of these strategies is that they are not brittle and do not tend to break whenever you push any update to the core infrastructure of the application. These are one of the best components if you want to implement full-stack strategies within your test environment.
Integration With Cloud Testing
While you are using these methods and practices to verify the proper functioning of all the input methods available with your application, you should also remember to verify the visual placement of all these fields:
Why is this important? Depending on the physical parameters like screen size or display resolution of your user’s device, these input fields might change their placement or, in a worst-case scenario, will not function completely.
We know that it is a very expensive and hectic process to set up and maintain a real device test lab. So we will suggest the best alternative to it, cloud testing platforms like LambdaTest.
LambdaTest is an AI-native test orchestration and execution platform that lets you perform manual and automation testing at scale with over 5000+ real devices, browsers, and OS combinations.
With it, you can easily integrate Selenium and Appium to initiate advanced processes like automated quality assurance testing, automated cross-browser testing, and automated web app testing.
Interested to learn more? The following code snippet will help you understand how you can integrate automated cross-browser testing with Selenium and LambdaTest:
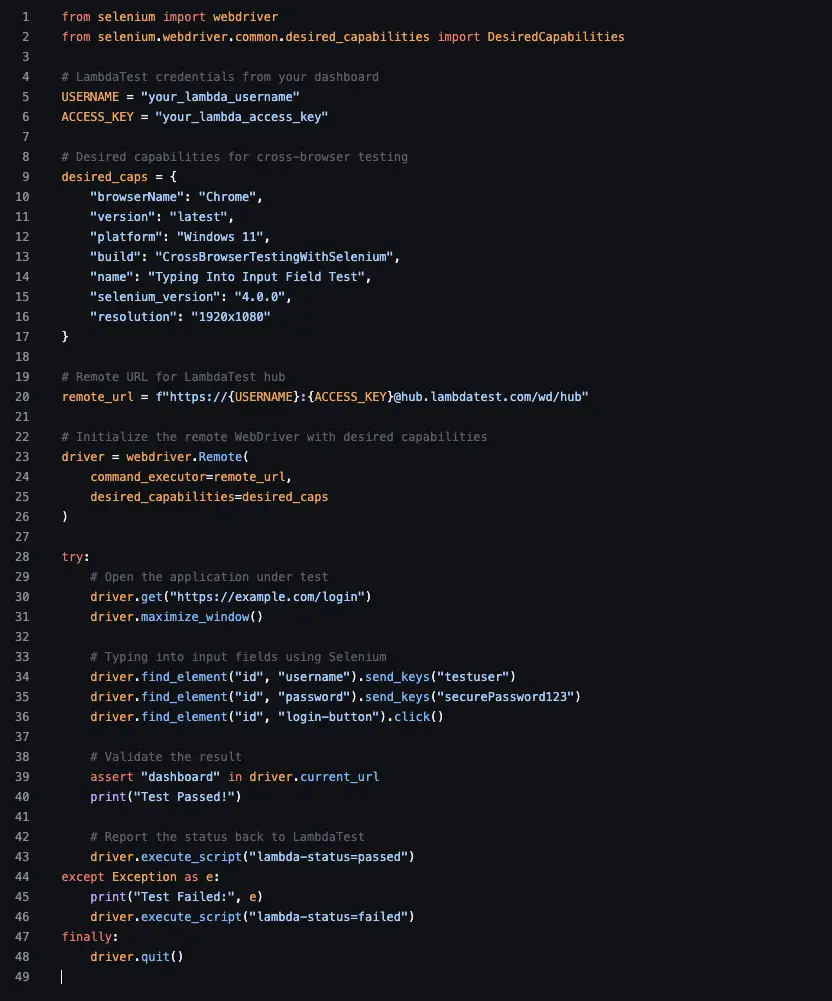
Best Practices To Improve Testing Reliability
We would strongly recommend that you incorporate the following practices within your testing workflow to ensure that your entire infrastructure is functioning smoothly:
- Before initiating any testing on the input fields, it is very important to wait for their visibility. Even if the element is present in the document object model, it may not be visible. So you can use the method “visibility_of_element_located” in this process.
- We’d strongly recommend that the testers use JavaScript executor to handle all the stubborn fields that might be present on your web application. These fields are usually those elements that cannot be easily intractable using the WebDriver method. To further help you understand, the following line of code will help achieve this goal:
driver.execute_script(“arguments[0].value=’admin’;”, input_field)
- We would strongly recommend that the testers have proper strategies to handle the focus and blur events that are present on almost all modern applications. This is because the apps rely on these elements to validate or trigger changes in the user interface. To implement this approach, you can simulate tabbing or clicking elsewhere to test how these elements are flowing.
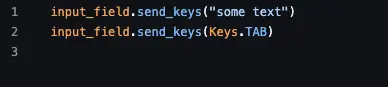
Apart from the above best practices, we would also encourage you to go through the official documentation of Selenium, where you will find more information about handling input fields with Selenium.
Challenges And Ways to Overcome Them
Like any other component of the modern testing process, you should also know that typing into input fields with Selenium will also have its own share of challenges.
However, with the right strategies and approach, you can easily overcome these challenges.
To shed more light on this segment, we have mentioned some of the major challenges with their most effective solutions:
- It is very common to face a situation when you will not be able to interact with the elements and input data within them. The most common solution is to wait for the visibility or use methods like ScrollIntoView or JavaScript Click to start interacting with these elements.
- If you encounter a scenario where your typing is being ignored or overwritten by the test environment, you can use the clear method first or the action class, and then start interacting with these elements.
- Suppose you have dynamic input elements that change their positioning or feature depending on user interactions, you have to wait for JavaScript or AJAX completion. Use explicit waits and then begin the testing process for these input fields.
- If you have a feature where the user can implement virtual keyboards or masked fields for interacting with your web application, you have to use JavaScript injection cautiously to ensure the dependability of the test cases.
- In certain cases, you will encounter a scenario where typing within the input fields will trigger the JavaScript validation process. To overcome this obstacle, you need to simulate blur or tap events after typing and then override the entire workflow.
Maintenance Tips
Apart from the solutions to overcome the most common challenges with input field testing with Selenium, we would also recommend that you implement the following maintenance tips to ensure that you are not facing challenges too often:
- You should always inspect the document object model for dynamic IDs and classes. Depending on all these elements, you should plan for them early and try to implement WebDriver wait methods.
- You should always attempt to abstract your locators and interactions into reusable functions. It is also very important to implement advanced design patterns like Page Object Models, which will help you to separate the test data from the test process. This process not only maintains the maintainability of the test cases but also helps improve their readability.
- We would always encourage you to document the keyboard sequences and actions if they differ depending on the browser that you’re using. We also recommend updating the WebDriver instances, like the Selenium ChromeDriver, to ensure the smoothness of the test execution process.
- Finally, you should use accessibility identifiers like “aria-* wherever possible to ensure that all your input methods are not only functioning properly but are also accessible by all users, irrespective of their possible disability.
The Bottom Line
Based on all the areas that we went through in this article, we can easily say that typing into input fields is one of the most frequently used functions of modern application testing. While certain static cases can be enough for common test scenarios, you will need to understand edge cases and real-world complexities to ensure that you are providing the best app user experience to your customers.
So, whether you are dealing with traditional input fields or advanced dynamic elements, all the best practices and strategies in our article will assist you in meeting all these requirements. We also suggest that you properly focus on improving the maintainability of these fields to ensure that they are viable in the long-term implementation.
Need one last advice? We recommend that you go through all the upcoming trends in this segment to ensure that you are including all the latest technologies and further elevating the experience of your application and building a positive reputation for your brand.